Have you ever run into this problem while developing?
The design calls for a headline with an emphasized word or tagline. This would be no problem when using the block editor, but what if you are designing a custom ACF block or ACF template and want the user to be able to easily apply alternative styling or emphasis to words within a simple text field?
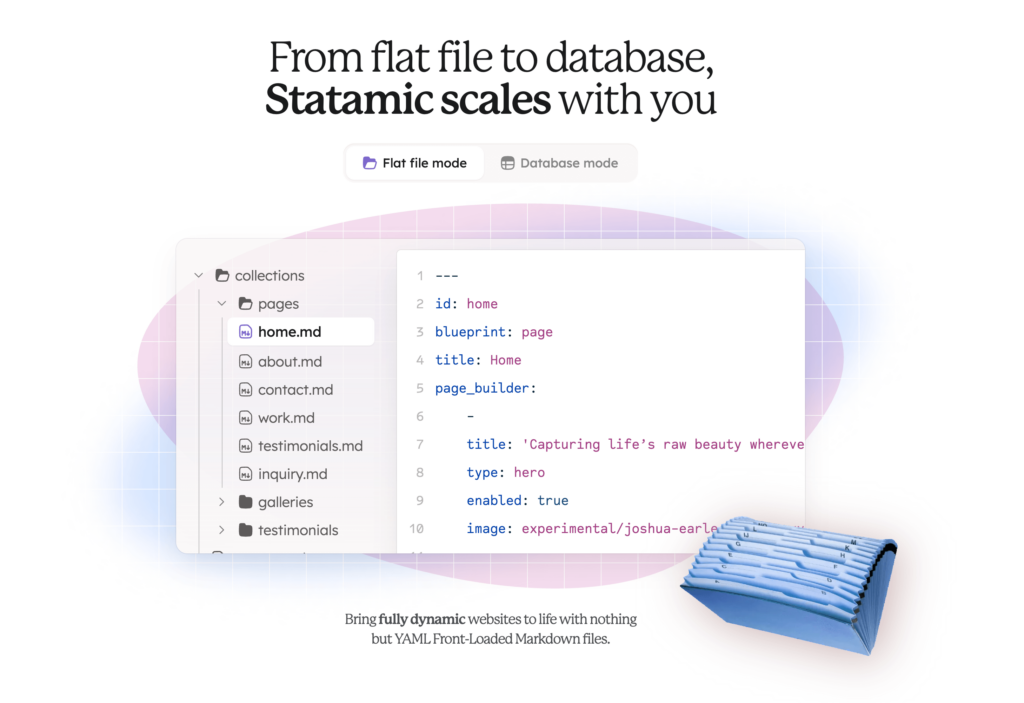
Markdown to the rescue
If you aren’t familiar with markdown, the idea is to provide a simple syntax for formatting within text documents. You can apply emphasis by wrapping words like **This** or a strong tag like ***so***. Many static site generators provide markdown processors to handle automatically converting markdown documents into html at build time.
We can use markdown as inspiration to make ACF fields more flexible. ACF provides a filter that processes the value saved within a field before outputting on the front end. We can hook into this, check what type of field we are dealing with, and conditionally apply our own markdown style transformations using Regex:
<?php
/**
* Allow markdown style formatting in ACF text fields
*/
function custom_acf_markdown_filters($value, $post_id, $field) {
// Check if the field type is 'text' and has a string value
if ($field['type'] === 'text' && is_string($value)) {
// IMPORTANT - ORDER MATTERS HERE
// add additional patterns and outputs as needed
$value = preg_replace('/\*\*(.*?)\*\*/', '<strong>$1</strong>', $value);
$value = preg_replace('/\*(.*?)\*/', '<em>$1</em>', $value);
}
return $value;
}
add_filter('acf/format_value', 'custom_acf_markdown_filters', 10, 3);
In the example above we have created logic to transform double and triple asterisks wrapped words into em and strong tags in the resulting HTML respectively (mirroring traditional markdown syntax).
Be sure to provide instructions to your users through the ACF field interface as well!
Once you have this setup, you can apply styling as needed based on these tags – you can go beyond just normal “strong” and “em” styling by scoping custom styles to the block or template where the field is used.
Going Further
While for simple tags like these I’d advise adhering to existing markdown conventions, you can also take this further and link special characters to custom classes as well.
For instance, lets pretend we have a site where certain words in a headlines have a squiggly underline treatment like so:
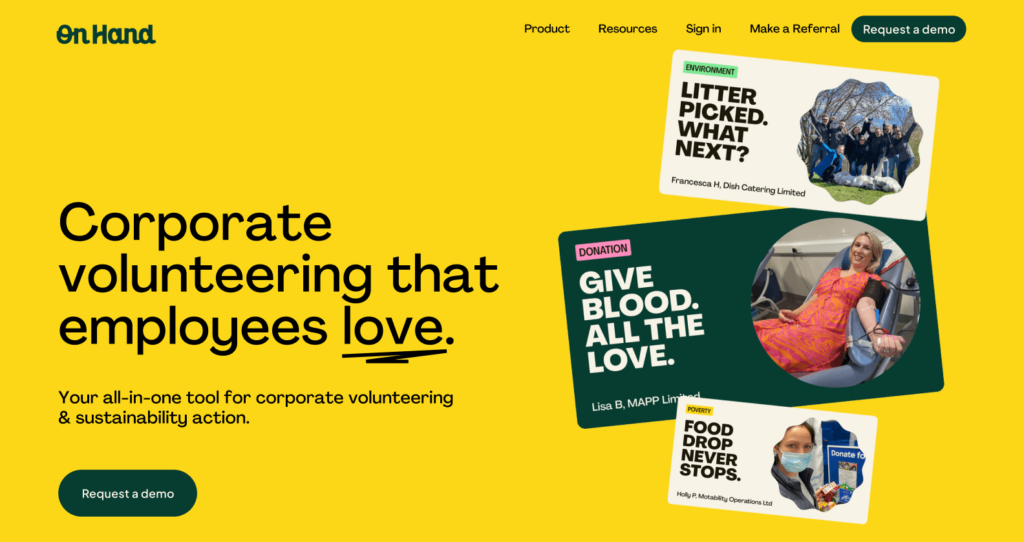
For me, I think the “~” symbol reminds me of the underline, so I can set up a simple filter to find words wrapped within these symbols and transform them to spans with a class of “underline–squiggly” or whatever semantic class name makes sense to you!
<?php
/**
* Allow markdown style formatting in ACF text fields
*/
function custom_acf_markdown_filters($value, $post_id, $field) {
// Check if the field type is 'text' and has a string value
if ($field['type'] === 'text' && is_string($value)) {
// add additional patterns and outputs as needed
$value = preg_replace('/\~(.*?)\~/', '<span class="underline--squiggly">$1</span>', $value);
}
return $value;
}
add_filter('acf/format_value', 'custom_acf_markdown_filters', 10, 3);
Now, in my text and text area fields I have some added functionality, without needing to resort to more complex solutions like a WYSIWYG editor, or needing to support inner blocks.
The end user can type something like this in the ACF text input:
Corporate volunteering that employees ~love.~
And get nice markup on the frontend with styling applied!
Wrapping up
I really love using this technique as it is both flexible and powerful.
You don’t want to overdo it with a ton of overwhelming options that your users will have trouble remembering, but for adding a few simple formatting options without messy toolbars, this approach really shines – especially if your end users are familiar with markdown syntax. Always ask, you’d be surprised how many non-technical people have been exposed to markdown at some point in their career.
Have you used this technique before? I’d love to hear about in the comments below.
Leave a Reply